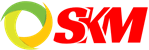
How do I make an HTTP request in Javascript?
You can make HTTP requests in JavaScript using the XMLHttpRequest
object or using the more modern fetch
API.
Here’s an example of using XMLHttpRequest
to make a GET request:
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onreadystatechange = function () {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send();
And here’s an example of using the fetch
API:
fetch('https://api.example.com/data')
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error(error));
Note that fetch
returns a Promise that resolves to the response object, which contains the response from the server. You can then use .text()
to get the response body as text. You can also use .json()
if the response from the server is in JSON format.